I’ve wanted to learn how to program for years, but I never got around to actually doing it. In this 3-part series, I’ll take you through the trials and tribulations of programming as a novice.
In the first article, “Looking for a language”, I went through the pros and cons of various languages, while in the second, “And the winner is…” I revealed my chosen language, JavaScript, and explained which resources I was going to use.
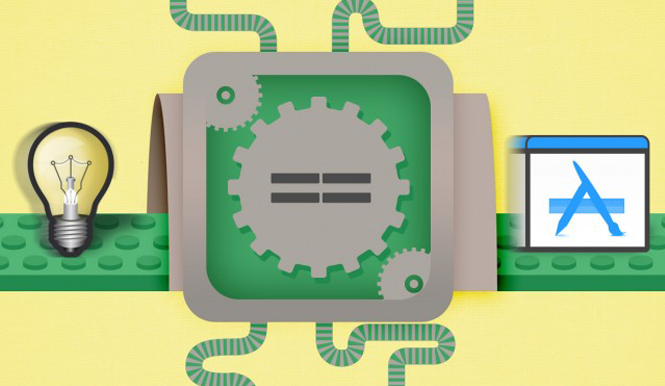
Now, it’s time to walk you through the first few steps you’ll take when you’re learning to program!
To refresh your memory: what you need to get started
As I explained in the last article, all you need to get started is a web browser and a text editor. My favorite browser is Chrome, which, even with default settings, has a fantastic set of web developer tools that you can check out by pressing F12.
These tools are all that you’ll need to get started with the language. Books, articles and interactive courses are all okay, but the only place to really dig your heels in, is on the actual web browser.
Opening the Chrome console
The Chrome console is where you can really try some coding. When you press F12 on your keyboard, the developer tools will appear in the bottom half of the window. Click on Console to see it.
To make things easier, undock the console from the main window; to do so, just click on the button in the bottom left-hand corner of the screen. You don’t need to have Chrome open, so you can minimize it.
The next step is to clear the console’s log history so you have a blank page. To do that, right click on the fourth button on the toolbar. You can do that as many times as you want to clear the console.
How does the Chrome console work?
Writing in the console is like having a conversation with your browser; you write a command, press Enter and the browser will answer you as quickly as possible. But in order to communicate, you have to be able to write in code, or more specifically, Javascript.
If you write something which isn’t code, this is what you will see:
The above photo shows a syntax error. The browser thinks that I’ve just written something which should be defined somewhere, but since it doesn’t understand the reference, it won’t respond. The problem: when you’re talking to a web browser, you have to spell everything out.
Try writing alert(“Hello, world”) and then pressing Enter. You’ll see a pop-up screen appear showing those words. It’s the most basic command there is, but as you can see, it works!
You might have noticed that just underneath the command, the word “undefined” appears. For now, don’t worry about that. It’s Chrome console’s way of saying that the code you’ve entered hasn’t returned a value (officially).
Basically though, that’s how the Chrome console works: you type text, press Enter and see the result.
Parts of a program: let’s get the blender out
A program takes in information, does something with it and gives back a result. In this sense, a program is like an invisible machine inside the computer, a machine made up of words that you enter.
To make this a bit clearer, I’m going to use a metaphor. Suppose we want to create a program called Blender that blends fruit down into delicious smoothies. It’s a silly example, but it’ll help you understand how computer programs work.
In its most basic form, the Blender program carries out four steps:
- Set up and turn on the blender
- Put fruit into the jug
- Blend the fruit into a smoothie
- Serve the smoothie in a glass
In the world of computers, nothing is taken for granted. You have to define everything in advance, including what we’re going to use and what operations must be carried out. So, what do we need to make this program work?
First of all, there’s the process of putting fruit into the blender, or jug. Next, there’s the actual blending of the fruit. Finally, there’s pouring the smoothie from the processor and serving it in a glass.
The “jug” and the “glass”, where we put the fruit we’re going to blend, are our variables; they are constants because we always have to put the fruit in the jug and pour the smoothie into the glass, no matter what we put in it. What changes, then, are the fruit: these ‘pieces’ of fruit will be our values.
The act of blending the fruit into a smoothie is the blender’s function, or at least one of its many functions. The function might incorporate conditions (such as ignore anything that isn’t fruit), or loops (blend the fruit down into a liquid).
Obviously, a computer program isn’t the same as a recipe, but the bare bones are the same: there is a series of steps to follow and repeat, some objects which alter other objects, and some conditions which must be fulfilled.
Content and containers: values and variables
In our metaphor, the blender blends fruit in a container (the jug). The container is the variable, and the content (fruit) is the value.
The data handled by a program is made up of values. In practice, those values can be either numbers or words. We use numbers to do calculations and words to communicate (inform, ask, say).
Essentially, JavaScript allows two basic (or primitive) types of values: numbers and strings of text. There are other types of special data, but we’ll come to them later; for the moment let’s focus on numbers and words.
In the console, you can enter numbers directly. For example, try writing the number 132399 in the Chrome console and press Enter. You’ll see that Chrome can only repeat this value back to you. It’s as if Chrome is saying “Roger that”.
Text chains, on the other hand, need quotation marks. Why? Because the instructions you use to create programs are also in words. The quotation marks tell the computer that what you’ve written is text.
In JavaScript, variables are established by writing “var” followed by the name you want to give to the variable (this is also called initializing the variable). Establishing these variables before using them is a good habit to get into, especially to avoid later confusion. Its almost like cooking- you want to have all the ingredients ready before you start.
When you put the variable in and identify it, the console will give you its current value.
JavaScript is pretty liberal when it comes to the naming variables, you just have to make sure that they begin with a letter of the alphabet and that there are no spaces. Your “containers” should be easily recognizable- it makes life easier for you and for the program.
Pour and compare: the many uses of =
As you can see, by initializing a variable (var = “Something”), you are using the equality operator =. In JavaScript, it has many different uses and meanings depending on the context. Every time you use =, it’s as if you are placing a tube for the value to travel through.
Note how the variable “Numerito” (number) changes value depending on what we tell the console. This is extremely flexible and useful when we want to update a variable while we are running a program.
But if = is used to assign values, then how then do we express equality? To do this, JavaScript has equality and comparison operators. The operator == checks that there is equality, while != does the same for inequality. Consider this example:
If the values are not equal or unequal, the answer is “false”; if they are equal, the answer is “true”. The same thing happens if we try to compare two values using the operator “greater than” and “less than”, along with “greater than or equal to”, and “less than or equal to”.
As you can see, it’s even possible to compare strings of text: the character order is based on standard Unicode, and JavaScript follows that Unicode to determine which letter comes before or after the other. In practice, it’s no more than an interesting detail.
Chop up and mix: arithmetic operators
Uploading your data is a good start, but just like you can’t make a bowl of pasta by simply putting the ingredients on the table, you can’t write a program without simple and clear instructions.
Using our food processor example, you have different settings such as chop, mix and blend. In JavaScript, which is one of the many languages your machine understands, the basic operations are the same as on a pocket calculator.
With numbers, you can do mathematical operations. Try writing a simple arithmetic operation in the console, such as 2 + 2, and press Enter. As you can see, the answer is 4.
Here, the + sign is an operator. In JavaScript, there are five main operators:
+ Add
– Subtract
* Multiply
/ Divide
% Modulus (division remainder)
All of these can be used exactly like they are on a calculator. Remember that JavaScript gives different importance to each operator when doing calculations. Thus, multiplication and division is done before subtraction and addition.
You can also use brackets to make things clearer. Another good habit to get into in terms of style is to put spaces between brackets, values, and operators. This makes your code easier to read.
There’s also one more thing you should know about operators: some can be used for more than one thing. In programming, this is called “operator overloading”.
Regarding the other main type of value, strings of text, you can use the operator + to join two or more strings of text together. This action is called “concatenation”, and it comes in handy when working with text.
For more complex things like inverting or deleting characters, you have to write your own custom functions. We’ll look at this later when we come to functions, blocks of code which you can use and reuse as many times as you want.
When using a real editor…
Until now, you have been writing on the Chrome console. That’s where you can keep trying out your code. But to create your own programs, it’s easier to use a conditional text editor, such as Sublime Text, which has syntax highlighting and automatic code formatting.
The syntax highlighting really helps with reading the code. Note, for example, that the text values appear in yellow, while the numbers are in purple. Also, Sublime Text automatically suggests words (variables, orders) to save time.
The most important thing, however, is the way in which Sublime Text formats code, adding tabs and skipping lines to improve the layout of your code, as well as making it more legible to you and other people reading it. The best thing about Sublime Text is that the editor does it all for you so that you can concentrate on the code itself.
The dark gray lines are comments. They are written with // at the start
While there is no such thing as a standard style guide to follow when writing in JavaScript, I recommend you write in a style you feel comfortable with and which is the clearest. I personally like writing in Idiomatic.js.
Exercises in Codecademy
Go to the JavaScript section of Codecademy and complete the unit titled “Getting Started with Programming”. You’ll find some things we’ll look at in the next chapter, but you’ll have no problem understanding it.
Conclusion: ingredients ready!
At this point, we’ve taken a look at all the basic ingredients of JavaScript programming: values, variables and operators. Next time, we’ll take a look at how to make the ‘food processor’ work, thanks to functions and loops.
Of course, if you’ve got any questions or doubts, just let me know in the comments!
Next time: functions and loops
Previous articles:
Learning to program 1 – looking for a language
Learning to program 2 – and the winner is…
Original article written by Fabrizio Ferri-Benedetti for Softonic ES.